Advanced Geocoding#
Overview#
Geocoding is the process of converting names of places into geographic coordinates.
Lets-Plot now offers geocoding API covering the following administrative levels:
country
state
county
city
Lets-Plot geocoding API allows a user to execute a single and batch geocoding queries, and handle possible names ambiguity.
The core class is Geocoder
. There is a function’s family for constructing the Geocoder
object - geocode_cities()
, geocode_counties()
, geocode_states()
, geocode_countries()
and geocode()
. For example:
1from lets_plot.geo_data import *
2countries = geocode_countries(['usa', 'canada'])
The geodata is provided by © OpenStreetMap contributors and is made available here under the Open Database License (ODbL).
get_xxx()
function is called. We will use in examples function get_geocodes()
which returns DataFrame
with metadata.Let us geocode countries. This code returns the DataFrame
object containing internal IDs for Canada and the US:
1countries.get_geocodes()
id | country | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 148838 | usa | United States | [-99.7426055742426, 37.2502586245537] | [-124.733375608921, 25.1162923872471, -66.9498... | [144.618412256241, -14.3740922212601, -64.5648... |
1 | 1428125 | canada | Canada | [-110.450525298983, 56.8387750536203] | [-141.002660393715, 41.6765552759171, -55.6205... | [-141.002660393715, 41.6765552759171, -52.6194... |
For example, this sample returns the DataFrame
object containing IDs of all cities matching “warwick”:
1geocode_cities('warwick') \
2 .allow_ambiguous() \
3 .get_geocodes()
id | city | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 119776 | warwick | Warwick | [-83.9205776783726, 31.8303624540567] | [-83.9291015267372, 31.8222776055336, -83.9120... | [-83.9291015267372, 31.8222776055336, -83.9120... |
1 | 176086 | warwick | Warwick | [-74.3590787617065, 41.2538411468267] | [-74.374563395977, 41.2334154546261, -74.33202... | [-74.374563395977, 41.2334154546261, -74.33202... |
2 | 176448 | warwick | Warwick | [-1.58227695103754, 52.3015402257442] | [-1.78017809987068, 52.2137045860291, -1.40608... | [-1.78017809987068, 52.2137045860291, -1.40608... |
3 | 181594 | warwick | Warwick | [-98.7057320814883, 47.8541030734777] | [-98.7164886295795, 47.8475135564804, -98.6948... | [-98.7164886295795, 47.8475135564804, -98.6948... |
4 | 184249 | warwick | Warwick | [-96.9995924696813, 35.6883452832699] | [-97.0261216163635, 35.6740544736385, -96.9776... | [-97.0261216163635, 35.6740544736385, -96.9776... |
5 | 4072420 | warwick | Warwick | [-81.8960721893947, 43.0157359689474] | [-82.0060113072395, 42.9303230345249, -81.7887... | [-82.0060113072395, 42.9303230345249, -81.7887... |
6 | 158818247 | warwick | Warwick | [-72.3365538645007, 42.667919844389] | [-72.4120393395424, 42.6094262301922, -72.2719... | [-72.4120393395424, 42.6094262301922, -72.2719... |
7 | 158863860 | warwick | Warwick | [-71.4332938210472, 41.715542525053] | [-71.5189133584499, 41.6628210246563, -71.3564... | [-71.5189133584499, 41.6293966770172, -71.3564... |
8 | 159726256 | warwick | Warwick | [-72.0051031618881, 45.952380001545] | [-72.0792764425278, 45.8764761686325, -71.9089... | [-72.0792764425278, 45.8764761686325, -71.9089... |
9 | 1817489924 | warwick | Warwick | [152.032703831792, -28.2163204997778] | [152.023720443249, -28.224236369133, 152.04168... | [152.023720443249, -28.224236369133, 152.04168... |
10 | 3049373 | warwick | Warwick Township | [-75.757813608352, 40.1801763474941] | [-75.8212745189667, 40.1465494930744, -75.6930... | [-75.8212745189667, 40.1465494930744, -75.6930... |
11 | 3521480 | warwick | Warwick Township | [-75.0764330968138, 40.2491855621338] | [-75.1225188374519, 40.2152167260647, -75.0345... | [-75.1225188374519, 40.2152167260647, -75.0345... |
12 | 9244563 | warwick | Warwick Mountain | [-63.3714760496144, 45.5978938937187] | [-63.4091444313526, 45.5644172430038, -63.3474... | [-63.4091444313526, 45.5644172430038, -63.3474... |
13 | 158903676 | warwick | West Warwick | [-71.5257788638961, 41.6969098895788] | [-71.5342850983143, 41.6620793938637, -71.4839... | [-71.5342850983143, 41.6620793938637, -71.4839... |
14 | 7997266 | warwick | Sainte-Élizabeth-de-Warwick | [-72.1010115992802, 45.9195195883512] | [-72.1493585407734, 45.8681344985962, -72.0435... | [-72.1493585407734, 45.8681344985962, -72.0435... |
DataFrame
object containing the ID of one particular “warwick” closest to Boston (US):1boston_us = geocode_cities('boston').scope('us')
2geocode_cities('warwick') \
3 .where('warwick', closest_to=boston_us) \
4 .get_geocodes()
id | city | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 158863860 | warwick | Warwick | [-71.4332938210472, 41.715542525053] | [-71.5189133584499, 41.6628210246563, -71.3564... | [-71.5189133584499, 41.6293966770172, -71.3564... |
Geocoder
object is available, it can be passed to any Lets-Plot geom supporting the map
parameter. map
parameter can be used to simply draw a GeoDataFrame or to draw a Geocoder. For more complex plots parameter map_join can be used to map data to geometries.If necessary, the Geocoder
object can be transformed to a geopandas GeoDataFrame
using one of get_centroids()
, get_boundaries()
, or get_limits()
methods.
All coordinates are in the EPSG:4326 coordinate reference system (CRS).
Note that an internet connection is required to execute geocoding queries.
Examples#
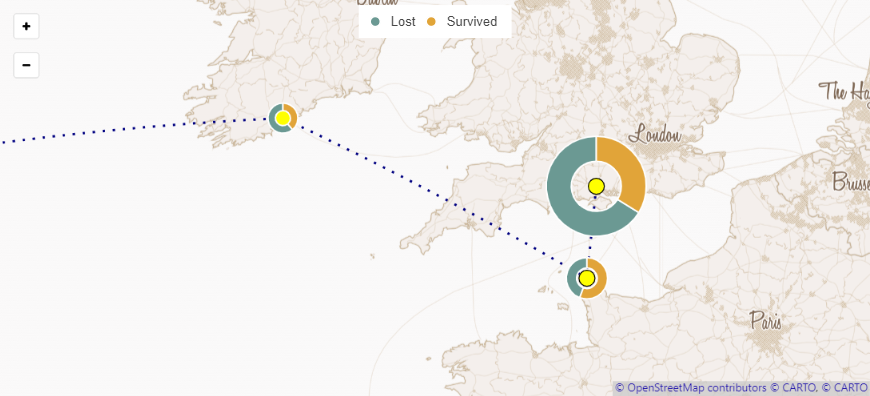
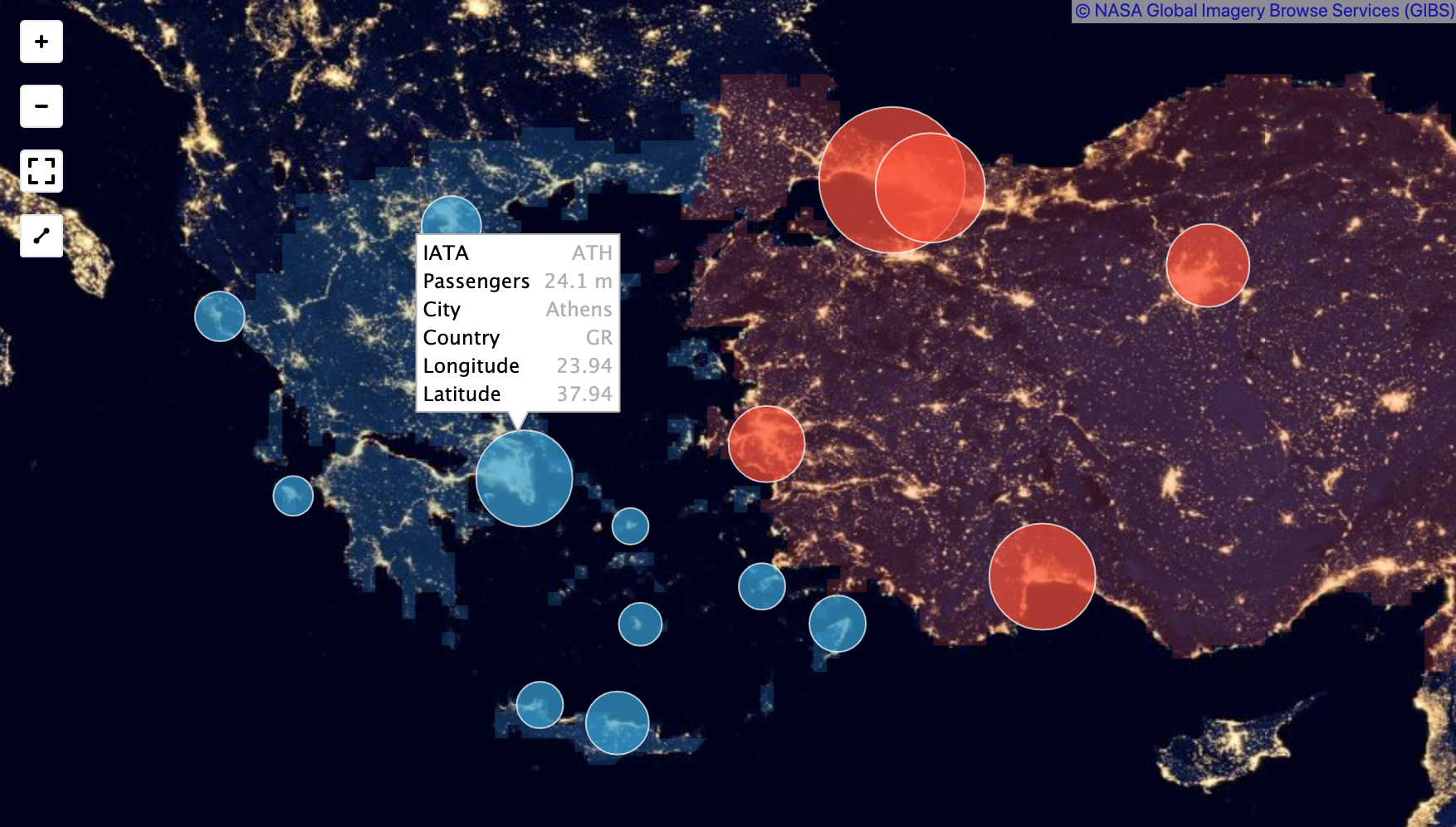
Reference#
The geocoding_reference.ipynb notebook contains a demonstration code covering use-cases presented in this section.
Levels#
Geocoding supports 4 administrative levels:
city
county
state
country
Function geocode()
with level=None
can try to detect level automatically - it enumerates all levels from country to city and selects best matching level (result without ambiguity and unknown names).
For example:
1geocode(names=['florida', 'tx']).get_geocodes()
id | state | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 162050 | florida | Florida | [-81.664617414276, 28.0571937561035] | [-87.6348964869976, 25.1162923872471, -80.0309... | [-87.6348964869976, 24.5230695605278, -80.0309... |
1 | 114690 | tx | Texas | [-99.6829525269137, 31.1685702949762] | [-106.645845472813, 25.8370596170425, -93.5078... | [-106.645845472813, 25.8370596170425, -93.5078... |
Functions geocode_cities()
, geocode_counties()
, geocode_states()
, geocode_countries()
or geocode(level=xxx)
search names only at a given level or return an error.
1geocode_states(['florida', 'tx']).get_geocodes()
id | state | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 162050 | florida | Florida | [-81.664617414276, 28.0571937561035] | [-87.6348964869976, 25.1162923872471, -80.0309... | [-87.6348964869976, 24.5230695605278, -80.0309... |
1 | 114690 | tx | Texas | [-99.6829525269137, 31.1685702949762] | [-106.645845472813, 25.8370596170425, -93.5078... | [-106.645845472813, 25.8370596170425, -93.5078... |
Parents#
Geocoder
class provides functions to define parents with administrative level - counties()
, states()
, countries()
.
These functions can handle single or multiple values of type string or Geocoder
. The number of values must match the number of names in Geocoder
so that they form a table.
Parents will be present in the result DataFrame
to make it possible to join data and geometry via map_join.
1geocode_cities(['warwick', 'worcester'])\
2 .counties(['Worth County', 'worcester county'])\
3 .states(['georgia', 'massachusetts'])\
4 .get_geocodes()
id | city | found name | county | state | centroid | position | limit | |
---|---|---|---|---|---|---|---|---|
0 | 119776 | warwick | Warwick | Worth County | georgia | [-83.9205776783726, 31.8303624540567] | [-83.9291015267372, 31.8222776055336, -83.9120... | [-83.9291015267372, 31.8222776055336, -83.9120... |
1 | 158851900 | worcester | Worcester | worcester county | massachusetts | [-71.8154652712922, 42.2678737342358] | [-71.8840424716473, 42.2100399434566, -71.7312... | [-71.8840424716473, 42.2100399434566, -71.7312... |
None
value for countries with different administrative division:1geocode_cities(['warwick', 'worcester'])\
2 .states(['Georgia', None])\
3 .countries(['USA', 'United Kingdom'])\
4 .get_geocodes()
id | city | found name | state | country | centroid | position | limit | |
---|---|---|---|---|---|---|---|---|
0 | 119776 | warwick | Warwick | Georgia | USA | [-83.9205776783726, 31.8303624540567] | [-83.9291015267372, 31.8222776055336, -83.9120... | [-83.9291015267372, 31.8222776055336, -83.9120... |
1 | 20971097 | worcester | Worcester | None | United Kingdom | [-2.2095610731112, 52.1965283900499] | [-2.2632023692131, 52.1616362035275, -2.157303... | [-2.2632023692131, 52.1616362035275, -2.157303... |
Geocoder
object. This allows resolving parent’s ambiguity:1s = geocode_states(['vermont', 'georgia']).scope('usa')
2geocode_cities(['worcester', 'warwick']).states(s).get_geocodes()
id | city | found name | state | centroid | position | limit | |
---|---|---|---|---|---|---|---|
0 | 8898137 | worcester | Worcester | vermont | [-72.5724501055639, 44.4132962822914] | [-72.6543393731117, 44.3454243242741, -72.4935... | [-72.6543393731117, 44.3454243242741, -72.4935... |
1 | 119776 | warwick | Warwick | georgia | [-83.9205776783726, 31.8303624540567] | [-83.9291015267372, 31.8222776055336, -83.9120... | [-83.9291015267372, 31.8222776055336, -83.9120... |
Scope#
scope()
is a special kind of parent.
scope()
can handle a string
or a single entry Geocoder
object.
scope()
is not associated with any administrative level, it acts as parent for any other parents and names.
scope()
can not be used with countries()
parents.
Typical use-case: all of names belong to the same parent.
1geocode_counties(['Dakota County', 'Nevada County']).states(['NE', 'AR']).scope('USA').get_geocodes()
id | county | found name | state | centroid | position | limit | |
---|---|---|---|---|---|---|---|
0 | 1425447 | Dakota County | Dakota County | NE | [-96.5715826334556, 42.4019493162632] | [-96.7274482548237, 42.2765184938908, -96.3566... | [-96.7274482548237, 42.2765184938908, -96.3566... |
1 | 1826825 | Nevada County | Nevada County | AR | [-93.2913903139467, 33.6979349702597] | [-93.4838207066059, 33.4403765201569, -93.1042... | [-93.4838207066059, 33.4403765201569, -93.1042... |
1florida = geocode_states('florida')
2
3display(florida.countries('usa').get_geocodes())
4display(florida.countries('uruguay').get_geocodes())
5display(florida.countries(None).get_geocodes())
id | state | found name | country | centroid | position | limit | |
---|---|---|---|---|---|---|---|
0 | 162050 | florida | Florida | usa | [-81.664617414276, 28.0571937561035] | [-87.6348964869976, 25.1162923872471, -80.0309... | [-87.6348964869976, 24.5230695605278, -80.0309... |
id | state | found name | country | centroid | position | limit | |
---|---|---|---|---|---|---|---|
0 | 1635164 | florida | Florida | uruguay | [-55.8642029687055, -33.7640165537596] | [-56.5363445878029, -34.4264329969883, -55.098... | [-56.5363445878029, -34.4264329969883, -55.098... |
id | state | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 162050 | florida | Florida | [-81.664617414276, 28.0571937561035] | [-87.6348964869976, 25.1162923872471, -80.0309... | [-87.6348964869976, 24.5230695605278, -80.0309... |
Fetch All#
It is possible to fetch all objects within parent - just do not set the names
parameter.
1geocode_counties().states('massachusetts').get_geocodes()
id | county | found name | state | centroid | position | limit | |
---|---|---|---|---|---|---|---|
0 | 61321 | Berkshire County | Berkshire County | massachusetts | [-73.1970960029264, 42.4073023349047] | [-73.5082098841667, 42.0398162305355, -72.9488... | [-73.5082098841667, 42.0398162305355, -72.9488... |
1 | 90434 | Essex County | Essex County | massachusetts | [-70.9657019377445, 42.6519144326448] | [-71.2561883032322, 42.4163138866425, -70.6729... | [-71.2561883032322, 42.4163138866425, -70.5907... |
2 | 1181619 | Hampden County | Hampden County | massachusetts | [-72.6295139278191, 42.1725875884295] | [-73.0750867724419, 41.9976854324341, -72.1350... | [-73.0750867724419, 41.9976854324341, -72.1350... |
3 | 1838804 | Hampshire County | Hampshire County | massachusetts | [-72.7141542430634, 42.369756102562] | [-73.0685086548328, 42.1833908557892, -72.2032... | [-73.0685086548328, 42.1833908557892, -72.2032... |
4 | 1838805 | Worcester County | Worcester County | massachusetts | [-71.9577156176713, 42.3648966103792] | [-72.3158794641495, 42.0080535113811, -71.4780... | [-72.3158794641495, 42.0080535113811, -71.4780... |
5 | 1839610 | Franklin County | Franklin County | massachusetts | [-72.5619375937952, 42.5192946195602] | [-73.0237090587616, 42.3032829165459, -72.2249... | [-73.0237090587616, 42.3032829165459, -72.2249... |
6 | 1840537 | Bristol County | Bristol County | massachusetts | [-71.1762539847584, 41.7951115965843] | [-71.3814635574818, 41.4945808053017, -70.8417... | [-71.3814635574818, 41.4809954166412, -70.8158... |
7 | 1840538 | Middlesex County | Middlesex County | massachusetts | [-71.2881300332954, 42.4460086226463] | [-71.8987718224525, 42.1567820012569, -71.0203... | [-71.8987718224525, 42.1567820012569, -71.0203... |
8 | 1840539 | Norfolk County | Norfolk County | massachusetts | [-71.2060019640526, 42.1540769934654] | [-71.503077596426, 41.9850830733776, -70.91473... | [-71.503077596426, 41.9850830733776, -70.78201... |
9 | 1840540 | Plymouth County | Plymouth County | massachusetts | [-70.8627991612476, 41.9707231968641] | [-71.0804834961891, 41.6237345337868, -70.5256... | [-71.0804834961891, 41.6237345337868, -70.5256... |
10 | 2294308 | Barnstable County | Barnstable County | massachusetts | [-69.9965259842594, 41.7987298965454] | [-70.686851888895, 41.5150675177574, -69.92929... | [-70.686851888895, 41.5150675177574, -69.92929... |
11 | 2298154 | Suffolk County | Suffolk County | massachusetts | [-71.0606561674246, 42.3390870541334] | [-71.1912493407726, 42.2279115021229, -70.9532... | [-71.1912493407726, 42.2279115021229, -70.9244... |
12 | 2387087 | Nantucket County | Nantucket County | massachusetts | [-70.0092203651615, 41.3159892708063] | [-70.2361777424812, 41.2393619120121, -69.9608... | [-70.3080931305885, 41.2393619120121, -69.9608... |
13 | 2387198 | Dukes County | Dukes County | massachusetts | [-70.6198368970106, 41.3932851701975] | [-70.838218331337, 41.3013222813606, -70.44638... | [-70.9499511122704, 41.2496776878834, -70.4463... |
US-48#
Geocoding supports a special name us-48
for CONUS.
The us-48
can be used as name or parent.
1geocode_states('us-48').get_geocodes()
id | state | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 60759 | Vermont | Vermont | [-72.772353529363, 43.8718488067389] | [-73.4377402067184, 42.7269606292248, -71.4653... | [-73.4377402067184, 42.7269606292248, -71.4653... |
1 | 61315 | Massachusetts | Massachusetts | [-72.0964509339039, 42.1913791447878] | [-73.5082098841667, 41.4945808053017, -69.9292... | [-73.5082098841667, 41.2393619120121, -69.9292... |
2 | 61320 | New York | New York | [-76.0912327538441, 42.8993669897318] | [-79.7619438171387, 40.7823456823826, -73.2414... | [-79.7619438171387, 40.4960802197456, -71.8561... |
3 | 63512 | Maine | Maine | [-69.1608471741827, 45.2623642981052] | [-71.0841688513756, 43.0649779736996, -66.9498... | [-71.0841688513756, 42.9808665812016, -66.9498... |
4 | 67213 | New Hampshire | New Hampshire | [-71.5517876605831, 44.0015134960413] | [-72.5572353601456, 42.6972283422947, -70.7018... | [-72.5572353601456, 42.6972283422947, -70.7018... |
5 | 114690 | Texas | Texas | [-99.6829525269137, 31.1685702949762] | [-106.645845472813, 25.8370596170425, -93.5078... | [-106.645845472813, 25.8370596170425, -93.5078... |
6 | 122586 | Illinois | Illinois | [-89.451321863232, 39.7387826442719] | [-91.5130515396595, 36.9701313972473, -87.0199... | [-91.5130515396595, 36.9701313972473, -87.0199... |
7 | 161638 | Missouri | Missouri | [-92.4924265655452, 38.3039845526218] | [-95.7741442322731, 35.9956835210323, -89.0988... | [-95.7741442322731, 35.9956835210323, -89.0988... |
8 | 161644 | Kansas | Kansas | [-98.328692575607, 38.5217376798391] | [-102.051756531, 36.9931246340275, -94.5882055... | [-102.051756531, 36.9931246340275, -94.5882055... |
9 | 161645 | Oklahoma | Oklahoma | [-97.21699429948, 35.3174138814211] | [-103.002461493015, 33.6191953718662, -94.4312... | [-103.002461493015, 33.6191953718662, -94.4312... |
10 | 161646 | Arkansas | Arkansas | [-92.4984827479225, 34.7509514540434] | [-94.6178559958935, 33.004105836153, -89.64224... | [-94.6178559958935, 33.004105836153, -89.64224... |
11 | 161648 | Nebraska | Nebraska | [-100.027538024238, 41.501209884882] | [-104.0535145998, 39.9999774992466, -95.308054... | [-104.0535145998, 39.9999774992466, -95.308054... |
12 | 161650 | Iowa | Iowa | [-93.1514127397129, 41.9395130127668] | [-96.6397158801556, 40.3756007552147, -90.1400... | [-96.6397158801556, 40.3756007552147, -90.1400... |
13 | 161652 | South Dakota | South Dakota | [-100.253885285003, 44.2193739116192] | [-104.057756513357, 42.4798929691315, -96.4363... | [-104.057756513357, 42.4798929691315, -96.4363... |
14 | 161653 | North Dakota | North Dakota | [-100.452325244499, 47.4677764624357] | [-104.049264639616, 45.9350354969501, -96.5543... | [-104.049264639616, 45.9350354969501, -96.5543... |
15 | 161655 | Kentucky | Kentucky | [-84.729118063289, 37.823773920536] | [-89.4172927737236, 36.497118473053, -81.96454... | [-89.5715090632439, 36.497118473053, -81.96454... |
16 | 161816 | Indiana | Indiana | [-86.1734508970078, 39.7625309228897] | [-88.0997018516064, 37.7717417478561, -84.7846... | [-88.0997018516064, 37.7717417478561, -84.7846... |
17 | 161838 | Tennessee | Tennessee | [-86.3173561038854, 35.8305955678225] | [-90.3102980554104, 34.982981979847, -81.64689... | [-90.3102980554104, 34.982981979847, -81.64689... |
18 | 161943 | Mississippi | Mississippi | [-89.7084286073575, 32.5858394801617] | [-91.6550087928772, 30.1744651794434, -88.0977... | [-91.6550087928772, 30.1744651794434, -88.0977... |
19 | 161950 | Alabama | Alabama | [-86.7421099329499, 32.6446247845888] | [-88.4731015563011, 30.2791893482208, -84.8882... | [-88.4731015563011, 30.2249671518803, -84.8882... |
20 | 161957 | Georgia | Georgia | [-83.2514879869572, 32.6792977005243] | [-85.6052421033382, 30.3557570278645, -80.8400... | [-85.6052421033382, 30.3557570278645, -80.8400... |
21 | 161961 | Colorado | Colorado | [-105.549469314711, 38.9999721944332] | [-109.060187637806, 36.9924259185791, -102.041... | [-109.060187637806, 36.9924259185791, -102.041... |
22 | 161991 | Wyoming | Wyoming | [-107.548611778195, 43.0007712543011] | [-111.055267006159, 40.9948222339153, -104.052... | [-111.055267006159, 40.9948222339153, -104.052... |
23 | 161993 | Utah | Utah | [-111.54915785543, 39.4988744705915] | [-114.052882343531, 36.9979673624039, -109.041... | [-114.052882343531, 36.9979673624039, -109.041... |
24 | 162014 | New Mexico | New Mexico | [-106.045169724866, 34.2040651291609] | [-109.050223231316, 31.3322111964226, -103.002... | [-109.050223231316, 31.3322111964226, -103.002... |
25 | 162018 | Arizona | Arizona | [-111.665190827228, 34.1682100296021] | [-114.818357974291, 31.3322138786316, -109.045... | [-114.818357974291, 31.3322138786316, -109.045... |
26 | 162050 | Florida | Florida | [-81.664617414276, 28.0571937561035] | [-87.6348964869976, 25.1162923872471, -80.0309... | [-87.6348964869976, 24.5230695605278, -80.0309... |
27 | 162061 | Ohio | Ohio | [-82.7062932155643, 40.3632525354624] | [-84.8203365504742, 38.4031417965889, -80.5189... | [-84.8203365504742, 38.4031417965889, -80.5189... |
28 | 162068 | West Virginia | West Virginia | [-80.2961093874269, 38.9198365062475] | [-82.644739151001, 37.2014826536179, -77.71902... | [-82.644739151001, 37.2014826536179, -77.71902... |
29 | 162069 | Washington, D.C. | Washington, D.C. | [-76.9879975677786, 38.8937935978174] | [-77.1197667717934, 38.7916302680969, -76.9093... | [-77.1197667717934, 38.7916302680969, -76.9093... |
30 | 162109 | Pennsylvania | Pennsylvania | [-77.7395653681049, 41.1167051643133] | [-80.5210827291012, 39.7197657823563, -74.6895... | [-80.5210827291012, 39.7197657823563, -74.6895... |
31 | 162110 | Delaware | Delaware | [-75.5803336262906, 39.1468942165375] | [-75.7890397310257, 38.4511278569698, -75.0505... | [-75.7890397310257, 38.4511278569698, -75.0505... |
32 | 162112 | Maryland | Maryland | [-75.9553945026409, 38.8234928995371] | [-79.4873057305813, 37.92381092906, -75.049993... | [-79.4873057305813, 37.9135474562645, -75.0499... |
33 | 162115 | Montana | Montana | [-109.343314037162, 46.6796220839024] | [-116.049231737852, 44.3579153716564, -104.039... | [-116.049231737852, 44.3579153716564, -104.039... |
34 | 162116 | Idaho | Idaho | [-115.464817458905, 45.4943814128637] | [-117.24303200841, 41.9880764186382, -111.0435... | [-117.24303200841, 41.9880764186382, -111.0435... |
35 | 165466 | Wisconsin | Wisconsin | [-89.7229295951697, 44.8979325592518] | [-92.889314442873, 42.4919508397579, -86.24954... | [-92.889314442873, 42.4919508397579, -86.24954... |
36 | 165471 | Minnesota | Minnesota | [-94.5024903450148, 46.4419361203909] | [-97.2392620146275, 43.4994287788868, -89.4833... | [-97.2392620146275, 43.4994287788868, -89.4833... |
37 | 165473 | Nevada | Nevada | [-116.666956541192, 38.5030842572451] | [-120.005727410316, 35.0018888711929, -114.039... | [-120.005727410316, 35.0018888711929, -114.039... |
38 | 165475 | California | California | [-119.994112927034, 37.277335524559] | [-124.409690648317, 32.5343263149261, -114.130... | [-124.409690648317, 32.5343263149261, -114.130... |
39 | 165476 | Oregon | Oregon | [-120.525597872899, 44.1131933033466] | [-124.56648722291, 41.9917939603329, -116.4635... | [-124.56648722291, 41.9917939603329, -116.4635... |
40 | 165479 | Washington | Washington | [-119.70860442837, 47.2730628401041] | [-124.733375608921, 45.5437226593494, -116.917... | [-124.755572229624, 45.5437226593494, -116.917... |
41 | 165789 | Michigan | Michigan | [-84.5068071817736, 45.0034050643444] | [-90.4186204075813, 41.6961273550987, -82.1228... | [-90.4186204075813, 41.6961273550987, -82.1228... |
42 | 165794 | Connecticut | Connecticut | [-72.6619366808976, 41.5181306004524] | [-73.7277755141258, 40.9867581725121, -71.7871... | [-73.7277755141258, 40.9867581725121, -71.7871... |
43 | 224040 | South Carolina | South Carolina | [-80.570954325977, 33.6225119233131] | [-83.353998363018, 32.0341077446938, -78.54161... | [-83.353998363018, 32.0341077446938, -78.54161... |
44 | 224042 | Virginia | Virginia | [-78.2939080535943, 38.0035275220871] | [-83.6753672361374, 36.5407902002335, -75.8670... | [-83.6753672361374, 36.5407902002335, -75.2452... |
45 | 224045 | North Carolina | North Carolina | [-79.0290157899437, 35.214960873127] | [-84.3218292295933, 33.8419796526432, -75.4610... | [-84.3218292295933, 33.8419796526432, -75.4610... |
46 | 224922 | Louisiana | Louisiana | [-92.5706733638148, 31.0122518241405] | [-94.0431873500347, 29.0049590170383, -88.9928... | [-94.0431873500347, 28.9292603731155, -88.8210... |
47 | 224951 | New Jersey | New Jersey | [-74.3697877915224, 40.1556817442179] | [-75.5598650872707, 38.9532735943794, -73.8940... | [-75.5598650872707, 38.9296942949295, -73.8940... |
48 | 392915 | Rhode Island | Rhode Island | [-71.6161388376275, 41.660745665431] | [-71.8864738941193, 41.3034854829311, -71.2255... | [-71.8864738941193, 41.1466526985168, -71.1203... |
Ambiguity#
Often geocoding can find multiple objects for a name or do not find anything. In this case error will be generated:
1geocode_cities(['warwick', 'worcester']).get_geocodes()
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
Cell In[14], line 1
----> 1 geocode_cities(['warwick', 'worcester']).get_geocodes()
File ~/Applications/miniconda3/envs/lets-plot-docs/lib/python3.10/site-packages/lets_plot/geo_data/geocoder.py:334, in Geocoder.get_geocodes(self)
315 def get_geocodes(self) -> 'DataFrame':
316 """
317 Return metadata for given regions.
318
(...)
332
333 """
--> 334 return self._geocode().to_data_frame()
File ~/Applications/miniconda3/envs/lets-plot-docs/lib/python3.10/site-packages/lets_plot/geo_data/geocoder.py:935, in NamesGeocoder._geocode(self)
933 response: Response = GeocodingService().do_request(request)
934 if not isinstance(response, SuccessResponse):
--> 935 _raise_exception(response)
936 self._geocodes = Geocodes(response.level, response.answers, request.region_queries, self._highlights)
938 return self._geocodes
File ~/Applications/miniconda3/envs/lets-plot-docs/lib/python3.10/site-packages/lets_plot/geo_data/geocodes.py:344, in _raise_exception(response)
342 def _raise_exception(response: Response):
343 msg = _format_error_message(response)
--> 344 raise ValueError(msg)
ValueError: Multiple objects (15) were found for warwick:
- Warwick (United States, Georgia, Worth County)
- Warwick (United States, New York, Orange County)
- Warwick (United Kingdom, England, West Midlands, Warwickshire)
- Warwick (United States, North Dakota, Benson County)
- Warwick (United States, Oklahoma, Lincoln County)
- Warwick (Canada, Ontario, Southwestern Ontario, Lambton County)
- Warwick (United States, Massachusetts, Franklin County)
- Warwick (United States, Rhode Island, Kent County)
- Warwick (Canada, Arthabaska, Québec, Centre-du-Québec)
- Warwick (Australia, Queensland)
Multiple objects (5) were found for worcester:
- Worcester (United States, Vermont, Washington County)
- Worcester (United Kingdom, England, West Midlands, Worcestershire)
- Worcester (South Africa, Western Cape, Cape Winelands District Municipality)
- Worcester (United States, Massachusetts, Worcester County)
- Worcester Township (United States, Pennsylvania, Montgomery County)
allow_ambiguous()
#
The best way is to find an object that we search and use its parents. The function converts error result into success result that can be rendered on a map or verified manually in other way. Can be combined with ignore_not_found() to suppress the “not found” error, which has higher priority.
1geocode_cities(['warwick', 'worcester']).allow_ambiguous().get_geocodes()
id | city | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 119776 | warwick | Warwick | [-83.9205776783726, 31.8303624540567] | [-83.9291015267372, 31.8222776055336, -83.9120... | [-83.9291015267372, 31.8222776055336, -83.9120... |
1 | 176086 | warwick | Warwick | [-74.3590787617065, 41.2538411468267] | [-74.374563395977, 41.2334154546261, -74.33202... | [-74.374563395977, 41.2334154546261, -74.33202... |
2 | 176448 | warwick | Warwick | [-1.58227695103754, 52.3015402257442] | [-1.78017809987068, 52.2137045860291, -1.40608... | [-1.78017809987068, 52.2137045860291, -1.40608... |
3 | 181594 | warwick | Warwick | [-98.7057320814883, 47.8541030734777] | [-98.7164886295795, 47.8475135564804, -98.6948... | [-98.7164886295795, 47.8475135564804, -98.6948... |
4 | 184249 | warwick | Warwick | [-96.9995924696813, 35.6883452832699] | [-97.0261216163635, 35.6740544736385, -96.9776... | [-97.0261216163635, 35.6740544736385, -96.9776... |
5 | 4072420 | warwick | Warwick | [-81.8960721893947, 43.0157359689474] | [-82.0060113072395, 42.9303230345249, -81.7887... | [-82.0060113072395, 42.9303230345249, -81.7887... |
6 | 158818247 | warwick | Warwick | [-72.3365538645007, 42.667919844389] | [-72.4120393395424, 42.6094262301922, -72.2719... | [-72.4120393395424, 42.6094262301922, -72.2719... |
7 | 158863860 | warwick | Warwick | [-71.4332938210472, 41.715542525053] | [-71.5189133584499, 41.6628210246563, -71.3564... | [-71.5189133584499, 41.6293966770172, -71.3564... |
8 | 159726256 | warwick | Warwick | [-72.0051031618881, 45.952380001545] | [-72.0792764425278, 45.8764761686325, -71.9089... | [-72.0792764425278, 45.8764761686325, -71.9089... |
9 | 1817489924 | warwick | Warwick | [152.032703831792, -28.2163204997778] | [152.023720443249, -28.224236369133, 152.04168... | [152.023720443249, -28.224236369133, 152.04168... |
10 | 3049373 | warwick | Warwick Township | [-75.757813608352, 40.1801763474941] | [-75.8212745189667, 40.1465494930744, -75.6930... | [-75.8212745189667, 40.1465494930744, -75.6930... |
11 | 3521480 | warwick | Warwick Township | [-75.0764330968138, 40.2491855621338] | [-75.1225188374519, 40.2152167260647, -75.0345... | [-75.1225188374519, 40.2152167260647, -75.0345... |
12 | 9244563 | warwick | Warwick Mountain | [-63.3714760496144, 45.5978938937187] | [-63.4091444313526, 45.5644172430038, -63.3474... | [-63.4091444313526, 45.5644172430038, -63.3474... |
13 | 158903676 | warwick | West Warwick | [-71.5257788638961, 41.6969098895788] | [-71.5342850983143, 41.6620793938637, -71.4839... | [-71.5342850983143, 41.6620793938637, -71.4839... |
14 | 7997266 | warwick | Sainte-Élizabeth-de-Warwick | [-72.1010115992802, 45.9195195883512] | [-72.1493585407734, 45.8681344985962, -72.0435... | [-72.1493585407734, 45.8681344985962, -72.0435... |
15 | 8898137 | worcester | Worcester | [-72.5724501055639, 44.4132962822914] | [-72.6543393731117, 44.3454243242741, -72.4935... | [-72.6543393731117, 44.3454243242741, -72.4935... |
16 | 20971097 | worcester | Worcester | [-2.2095610731112, 52.1965283900499] | [-2.2632023692131, 52.1616362035275, -2.157303... | [-2.2632023692131, 52.1616362035275, -2.157303... |
17 | 30670038 | worcester | Worcester | [19.4459268450737, -33.6462374031544] | [19.4369441270828, -33.6537154018879, 19.45490... | [19.4369441270828, -33.6537154018879, 19.45490... |
18 | 158851900 | worcester | Worcester | [-71.8154652712922, 42.2678737342358] | [-71.8840424716473, 42.2100399434566, -71.7312... | [-71.8840424716473, 42.2100399434566, -71.7312... |
19 | 3076291 | worcester | Worcester Township | [-75.3438698875367, 40.1926231384277] | [-75.4107637703419, 40.1558580994606, -75.2932... | [-75.4107637703419, 40.1558580994606, -75.2932... |
ignore_not_found()
#
Removes unknown names from the result.
1geocode_cities(['paris', 'foo']).ignore_not_found().get_geocodes()
id | city | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 17807753 | paris | Paris | [2.32002815231681, 48.8587861508131] | [2.22412258386612, 48.8155750930309, 2.4697606... | [2.22412258386612, 48.8155750930309, 2.4697606... |
ignore_all_errors()
#
Remove not found names or names with multiple matches.
1geocode_cities(['paris', 'worcester', 'foo']).ignore_all_errors().get_geocodes()
id | city | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 17807753 | paris | Paris | [2.32002815231681, 48.8587861508131] | [2.22412258386612, 48.8155750930309, 2.4697606... | [2.22412258386612, 48.8155750930309, 2.4697606... |
where()
#
For resolving an ambiguity geocoding provides a function that can configure names individually.
To configure a name the function where(...)
should be called with the place name and all used parent names.
Parents cannot be changed via where()
function call.
If name and parents do not match with ones from the where()
function an error will be generated.
It is important for cases like this:
1geocode_counties(['Washington', 'Washington']).states(['oregon', 'utah']).get_geocodes()
id | county | found name | state | centroid | position | limit | |
---|---|---|---|---|---|---|---|
0 | 1837133 | Washington | Washington County | oregon | [-123.09834180777, 45.5489509552717] | [-123.486059904099, 45.3171966969967, -122.743... | [-123.486059904099, 45.3171966969967, -122.743... |
1 | 1744372 | Washington | Washington County | utah | [-113.476485065118, 37.3094066977501] | [-114.052882343531, 37.0000769197941, -112.899... | [-114.052882343531, 37.0000769197941, -112.899... |
With parameter closest_to
geocoding will take only the object closest to given place.
Parameter closest_to
can be a single value Geocoder
.
1boston = geocode_cities('boston')
2geocode_cities('worcester').where('worcester', closest_to=boston).get_geocodes()
id | city | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 158851900 | worcester | Worcester | [-71.8154652712922, 42.2678737342358] | [-71.8840424716473, 42.2100399434566, -71.7312... | [-71.8840424716473, 42.2100399434566, -71.7312... |
shapely.geometry.Point
.1import shapely
2
3geocode_cities('worcester').where('worcester', closest_to=shapely.geometry.Point(-71.088, 42.311)).get_geocodes()
id | city | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 158851900 | worcester | Worcester | [-71.8154652712922, 42.2678737342358] | [-71.8840424716473, 42.2100399434566, -71.7312... | [-71.8840424716473, 42.2100399434566, -71.7312... |
With parameter scope
a shapely.geometry.Polygon
can be used for limiting an area of the search (coordinates should be in WGS84 coordinate system).
Note that bbox of the polygon will be used:
1geocode_cities('worcester')\
2 .where('worcester', scope=shapely.geometry.box(-71.00, 42.00, -72.00, 43.00))\
3 .get_geocodes()
id | city | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 158851900 | worcester | Worcester | [-71.8154652712922, 42.2678737342358] | [-71.8840424716473, 42.2100399434566, -71.7312... | [-71.8840424716473, 42.2100399434566, -71.7312... |
scope
can be a single value Geocoder
object or a string
:1massachusetts = geocode_states('massachusetts')
2geocode_cities('worcester').where('worcester', scope=massachusetts).get_geocodes()
id | city | found name | centroid | position | limit | |
---|---|---|---|---|---|---|
0 | 158851900 | worcester | Worcester | [-71.8154652712922, 42.2678737342358] | [-71.8840424716473, 42.2100399434566, -71.7312... | [-71.8840424716473, 42.2100399434566, -71.7312... |
scope
does not change parents in the result DataFrame
:1worcester_county=geocode_counties('Worcester County').states('massachusetts').countries('usa')
2
3geocode_cities(['worcester', 'worcester'])\
4 .countries(['USA', 'United Kingdom'])\
5 .where('worcester', country='USA', scope=worcester_county)\
6 .get_geocodes()
id | city | found name | country | centroid | position | limit | |
---|---|---|---|---|---|---|---|
0 | 158851900 | worcester | Worcester | USA | [-71.8154652712922, 42.2678737342358] | [-71.8840424716473, 42.2100399434566, -71.7312... | [-71.8840424716473, 42.2100399434566, -71.7312... |
1 | 20971097 | worcester | Worcester | United Kingdom | [-2.2095610731112, 52.1965283900499] | [-2.2632023692131, 52.1616362035275, -2.157303... | [-2.2632023692131, 52.1616362035275, -2.157303... |
Working with Plots#
Plotting a GeoDataFrame
#
get_xxx()
functions return GeoDataFrame which can be used as data
or map
parameter (see this or this).
1from lets_plot import *
2LetsPlot.setup_html()
3
4ggplot() + geom_point(map=geocode_states('us-48').get_centroids())
Plotting a Geocoder
#
Drawing geometries with Geocoder
is a bit easier than using GeoDataFrame
.
Just pass a Geocoder
to the map
parameter, and the layer will fetch geometry it supports:
1ggplot() + geom_point(map=geocode_states('us-48'))
geom_point(), geom_text() |
get_centroids() |
geom_map(), geom_polygon() |
get_boundaries() |
geom_rect() |
get_limits() |
map
and map_join
#
Parameter map_join
is used to join map coordinates with data. Map join is expected to be a str
, list[str]
or list[list[str]]
.
first value in a pair is a data_key (column/columns in
data
),second value in a pair is a map_key (column/columns in
map
).
Join with GeoDataFrame
#
Explicitly set keys for both data and map.
map_join=['data_column', 'map_column']
: same as[['data_column'], ['map_column']]
map_join=[['data_column_1', 'data_column_2'], ['map_column_1', 'map_column_2']]
: same as[['data_column_1', 'data_column_2'], ['map_column_1', 'map_column_2']]
map_join=[['City_Name', 'State_Name'], ['city', 'state']]
:
Single string key is not allowed - Lets-Plot can’t deduce a map key on a user generated GeoDataFrames.
Join with Geocoder
#
Geocoder
and GeoDataFrame
, returned by a Geocoder
geometries fetching functions, contains metadata so in most cases only data keys have to be provided - Lets-Plot will generate map keys automatically with columns that were used for geocoding.
map_join='State_Name'
: same as[['State_Name'], ['state']]
map_join=[['City_Name', 'State_Name']]
: same as[['City_Name', 'State_Name'], ['city', 'state']]
map_join=[['City_Name', 'State_Name'], ['city', 'state']]
: Explicitly set keys for both data and map.
Note
Generated keys follow this order - city
, county
, state
, country
.
Parents that were not provided will be omitted.
Data columns should follow the same order or result of join operation will be incorrect.